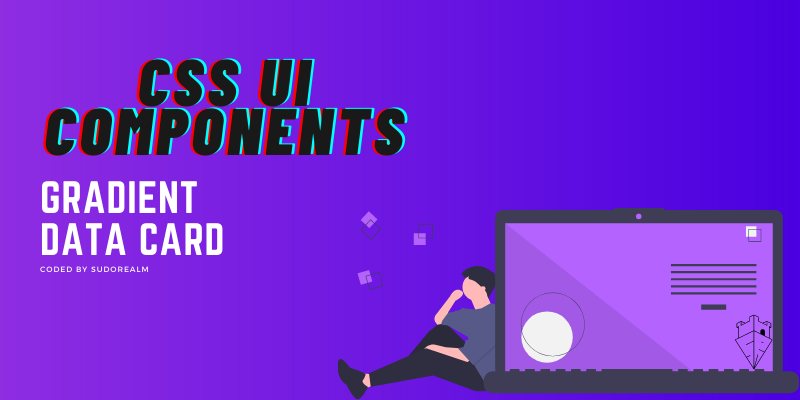
CSS Gradient Cards for Data Display
— 7 min read
Hello to all the cool coders out there! Welcome to the first of many coding tutorials of Sudorealm! My name is Thanos and I am an Engineer, a web developer, and a coder overall!
In this tutorial, you are gonna create the following!
How cool is that? Let's dive in.
For the skilled coder - The Code
The Code in a fiddle! Enjoy!
The Tools:
For the curious starter - The tutorial
This little UI Component was coded with pure HTML, some basic CSS, and the help of the Bootstrap Framework working together with the Font Awesome 5 popular icon set and toolkit.
Adding Bootstrap Starter Template
Nothing special here, just open up your index.html and paste the following code!
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous">
<title>Hello, world!</title>
</head>
<body>
<h1>Hello, world!</h1>
<!-- Optional JavaScript -->
<!-- jQuery first, then Popper.js, then Bootstrap JS -->
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="sha384-JjSmVgyd0p3pXB1rRibZUAYoIIy6OrQ6VrjIEaFf/nJGzIxFDsf4x0xIM+B07jRM" crossorigin="anonymous"></script>
</body>
</html>
Good, now you are ready to code an entire website's front end with the help of Bootstrap. But you need some icons.. right? Icons help with giving to the user a better understanding of what he/she is about to click. 👍
Adding Font Awesome Icon Pack to the Game!
This is a very easy part as well, just add on the <head></head> section of your code this beautiful line:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css">
And you are set! You have access to the large free icon pack of font-awesome!
The HTML body
The following code is a mixture of simple HTML, bootstrap classes, and some custom CSS classes that I will provide to you in the next section.
Place all of this code inside the <body></body>
<div class="container bg-gray pt-4 pb-5">
<h3 class="font-weight-bold mb-0">
Gradient Cards With Data
</h3>
<p class='text-uppercase text-muted'>
Beautiful Gradient Cards for showcasing different kinds of data
</p>
<div class="row">
<div class="col-xl-3 col-md-3 col-sm-6">
<div class="card mb-3 post-card text-left">
<a class="decoration-none" href="#">
<div class="card-body text-white-secondary bg-gr-purple">
<h4 class="card-title font-main mb-0">#Title 1</h4>
<p class="font-important-stat text-white mb-0">12
<span class="font-secondary">
<i class="fas fa-gem " aria-hidden="true"></i>
</span>
</p>
<p class="text-white-secondary mb-0">Posts</p>
</div>
</a>
</div>
</div>
<div class="col-xl-3 col-md-3 col-sm-6">
<div class="card mb-3 post-card text-left">
<a class="decoration-none" href="#">
<div class="card-body text-white-secondary bg-gr-blue">
<h4 class="card-title font-main mb-0">#Title 2</h4>
<p class="font-important-stat text-white mb-0">5
<span class="font-secondary">
<i class="fas fa-cube" aria-hidden="true"></i>
</span>
</p>
<p class="text-white-secondary mb-0">Categories</p>
</div>
</a>
</div>
</div>
<div class="col-xl-3 col-md-3 col-sm-6">
<div class="card mb-3 post-card text-left">
<a class="decoration-none" href="#">
<div class="card-body text-white-secondary bg-gr-green">
<h4 class="card-title font-main mb-0">#Title 3</h4>
<p class="font-important-stat text-white mb-0">68
<span class="font-secondary">
<i class="fas fa-user-friends" aria-hidden="true"></i>
</span>
</p>
<p class="text-white-secondary mb-0">Users</p>
</div>
</a>
</div>
</div>
<div class="col-xl-3 col-md-3 col-sm-6">
<div class="card mb-3 post-card text-left">
<a class="decoration-none" href="#">
<div class="card-body text-white-secondary bg-gr-red">
<h4 class="card-title font-main mb-0">#Title 4</h4>
<p class="font-important-stat text-white mb-0">419
<span class="font-secondary">
<i class="fas fa-heart" aria-hidden="true"></i>
</span>
</p>
<p class="text-white-secondary mb-0">Likes</p>
</div>
</a>
</div>
</div>
</div>
<p class="text-uppercase text-secondary float-right">Coded by
<a href='https://sudorealm.com' target='_blank'>Sudorealm.com</a></p>
</div>
In this code, you see classes like text-white, card, card-body, text-uppercase etc... Which are pretty much self-explanatory.
But you also see classes like col-xl-3, col-md-3, and col this col that. Well, col stands for column and it's a cool Bootstrap technique to keep your UI organized!
Or as they call it The Bootstrap Grid. It is a must to master! When you finally get the hang of it you will be able to construct mobile-friendly UIs in no time!
The styling part
While Bootstrap takes care of all the important stuff, like positioning, mobile responsiveness, and some text styling, in order to complete our Gradient card UI we need to include some custom classes.
In my humble opinion, a web designer should always get dirty with his/her CSS. I know that nowadays Frameworks like Bootstrap, Bulma, and Tailwind can create a professionally looking website without even needing to write down a single line of custom CSS, but, you know... The output of these Frameworks is pretty much the same every time. You can change colors and text sizes but in the end, you will always be like. Oh... that's a Bootstrap Website...
All I am trying to say is, get your hands a little dirty and try to give your websites a small touch of yourself! 😊
Ok! To the point! The following code is the custom CSS styling i used to create the Gradient Cards.
body{
font-family: 'PT Sans', sans-serif;
}
.text-white-secondary{
color: hsla(0, 0%, 100%, 0.7)
}
.font-main{
font-size: 18px;
}
.font-important-stat{
font-size: 40px;
font-weight: 700;
}
.font-secondary{
font-size:16px;
}
.bg-gray{
background-color: #f5f5fB;
}
.decoration-none{
text-decoration: none !important;
}
.bg-gr-purple{
background: #8E2DE2; /* fallback for old browsers */
background: -webkit-linear-gradient(to right, #4A00E0, #8E2DE2); /* Chrome 10-25, Safari 5.1-6 */
background: linear-gradient(to right, #4A00E0, #8E2DE2); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
}
.bg-gr-blue{
background: #0052D4; /* fallback for old browsers */
background: -webkit-linear-gradient(to left, #6FB1FC, #4364F7, #0052D4); /* Chrome 10-25, Safari 5.1-6 */
background: linear-gradient(to left, #6FB1FC, #4364F7, #0052D4); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
}
.bg-gr-green{
background: #00b09b; /* fallback for old browsers */
background: -webkit-linear-gradient(to left, #96c93d, #00b09b); /* Chrome 10-25, Safari 5.1-6 */
background: linear-gradient(to left, #96c93d, #00b09b); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
}
.bg-gr-red{
background: #ED213A; /* fallback for old browsers */
background: -webkit-linear-gradient(to right, #93291E, #ED213A); /* Chrome 10-25, Safari 5.1-6 */
background: linear-gradient(to right, #93291E, #ED213A); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
}
.post-card{
box-shadow: none;
}
.post-card:hover{
box-shadow: 0 2px 5px 0 rgba(0,0,0,0.16), 0 2px 10px 0 rgba(0,0,0,0.12);
}
A big shoutout to uigradients.com for their perfect website! I always use it when I go hunting for cool Gradient backgrounds!
You can either Embed CSS by adding all the code inside <style></style> tags on the <head> part of the code or add the styling by linking a CSS external file like so:
<link rel="stylesheet" href="pathto/styles.css" type="text/css">
This also goes in the <head> tag. 🤓
That was the last step, easy as riding an e-scooter! I am sure that you are about to create something extraordinary!
Conclusion
The code is as simple as it gets! But it is a good starting point I think. I really love coding, and I also love giving back to the community, so this is just the beginning of a journey for me. Stay Tuned! 📻
If this tutorial helped you send us a message on our Facebook Page we would love to chat with you about anything nerdy, we are the nerdiest realm after all, and if you really really like this type of content why don't like our page while you are at it! 😍
You can show your support by Signing In to the nerdiest realm of the internet
Oh, last but not least! If you are one of those super cool guys that really like to hype people up with crazy acts of kindness
(a really cold espresso is what kickstarts my whole day)
I'd like to thank you for taking the time to read one of my articles, you are the best!
Until the next one, my name is Thanos and I am... OUT 💨